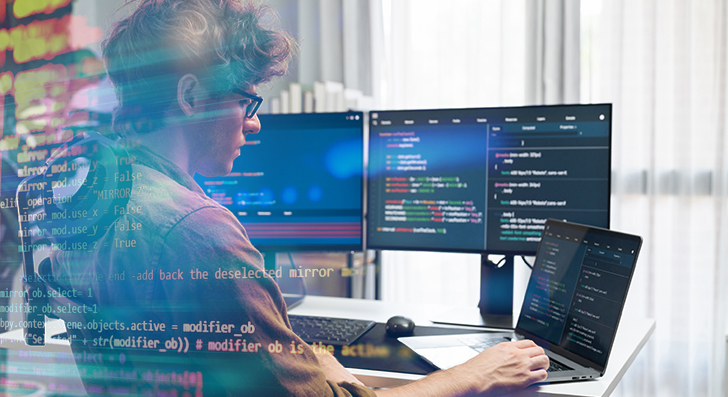
Scalability usually means your software can tackle advancement—far more consumers, more details, plus more targeted traffic—without having breaking. As being a developer, setting up with scalability in mind will save time and pressure afterwards. Listed here’s a transparent and functional manual to help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on later—it ought to be part of your respective strategy from the start. Numerous apps fail whenever they increase fast due to the fact the original layout can’t handle the extra load. For a developer, you have to Assume early about how your procedure will behave under pressure.
Start off by designing your architecture to get adaptable. Steer clear of monolithic codebases wherever everything is tightly linked. Rather, use modular layout or microservices. These styles break your app into more compact, independent elements. Just about every module or company can scale on its own without the need of affecting The entire technique.
Also, give thought to your databases from day 1. Will it need to have to take care of one million end users or merely 100? Pick the right sort—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t need to have them nonetheless.
A further important stage is to prevent hardcoding assumptions. Don’t compose code that only performs beneath recent ailments. Consider what would occur In case your user base doubled tomorrow. Would your app crash? Would the database slow down?
Use design styles that support scaling, like concept queues or occasion-driven methods. These assist your application tackle extra requests without the need of finding overloaded.
Any time you build with scalability in your mind, you are not just getting ready for success—you're reducing upcoming complications. A properly-planned system is easier to maintain, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Selecting the right databases is usually a critical Portion of developing scalable purposes. Not all databases are designed precisely the same, and using the Completely wrong you can slow you down or maybe induce failures as your application grows.
Begin by being familiar with your knowledge. Is it really structured, like rows within a table? If Of course, a relational database like PostgreSQL or MySQL is a great healthy. These are generally powerful with interactions, transactions, and consistency. In addition they assistance scaling procedures like go through replicas, indexing, and partitioning to take care of a lot more traffic and data.
When your data is much more adaptable—like consumer exercise logs, item catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at managing big volumes of unstructured or semi-structured facts and can scale horizontally a lot more easily.
Also, take into account your browse and create designs. Are you carrying out lots of reads with less writes? Use caching and browse replicas. Are you handling a weighty generate load? Consider databases that will cope with high create throughput, or simply event-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Consider in advance. You might not need Sophisticated scaling options now, but deciding on a databases that supports them means you won’t require to switch later on.
Use indexing to speed up queries. Keep away from unneeded joins. Normalize or denormalize your facts based upon your accessibility patterns. And usually check database functionality while you increase.
Briefly, the appropriate databases will depend on your application’s composition, velocity desires, And exactly how you hope it to mature. Choose time to select correctly—it’ll preserve plenty of problems later.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, just about every modest delay adds up. Improperly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial to Construct effective logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away anything at all unnecessary. Don’t pick the most intricate Option if a simple one will work. Maintain your functions small, targeted, and straightforward to check. Use profiling tools to uncover bottlenecks—spots exactly where your code will take too very long to run or works by using a lot of memory.
Next, have a look at your database queries. These typically slow points down greater than the code alone. Be sure Every question only asks for the info you really have to have. Stay away from Find *, which fetches every little thing, and in its place pick unique fields. Use indexes to speed up lookups. And prevent performing a lot of joins, Particularly throughout significant tables.
Should you detect the same knowledge remaining requested over and get more info over, use caching. Retail outlet the results temporarily applying resources like Redis or Memcached and that means you don’t really have to repeat costly functions.
Also, batch your databases functions when you can. As opposed to updating a row one by one, update them in teams. This cuts down on overhead and will make your application more successful.
Make sure to exam with large datasets. Code and queries that perform wonderful with one hundred data could possibly crash when they have to handle 1 million.
In brief, scalable apps are quickly applications. Keep the code limited, your queries lean, and use caching when needed. These steps assist your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more users and even more targeted traffic. If almost everything goes by way of just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching are available in. These two tools help keep the application rapidly, secure, and scalable.
Load balancing spreads incoming website traffic throughout a number of servers. As opposed to one particular server carrying out each of the function, the load balancer routes users to distinctive servers based upon availability. What this means is no single server receives overloaded. If just one server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for exactly the same information and facts yet again—like an item web page or simply a profile—you don’t ought to fetch it in the databases each and every time. You can provide it in the cache.
There's two frequent different types of caching:
1. Server-facet caching (like Redis or Memcached) merchants data in memory for speedy entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files close to the person.
Caching minimizes databases load, improves pace, and will make your app additional effective.
Use caching for things which don’t modify normally. And often be certain your cache is up to date when facts does alter.
Briefly, load balancing and caching are simple but strong applications. With each other, they assist your application handle far more buyers, keep speedy, and recover from troubles. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable apps, you would like tools that let your app increase quickly. That’s where cloud platforms and containers come in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t must get components or guess long run ability. When website traffic improves, you are able to include a lot more assets with only a few clicks or instantly employing automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your app rather than managing infrastructure.
Containers are another vital Software. A container deals your app and every little thing it must run—code, libraries, configurations—into one particular unit. This makes it quick to maneuver your app between environments, from a laptop computer towards the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes assist you to regulate them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of your respective app crashes, it restarts it quickly.
Containers also help it become easy to different elements of your application into products and services. It is possible to update or scale components independently, which happens to be great for general performance and dependability.
In short, employing cloud and container tools suggests you'll be able to scale speedy, deploy very easily, and Get better swiftly when complications take place. If you prefer your app to improve with out boundaries, commence applying these resources early. They help save time, reduce chance, and help you remain centered on setting up, not fixing.
Keep an eye on All the things
Should you don’t watch your software, you won’t know when items go Erroneous. Checking assists you see how your application is carrying out, place difficulties early, and make improved decisions as your app grows. It’s a critical Element of developing scalable techniques.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your app as well. Keep watch over just how long it requires for end users to load web pages, how frequently problems come about, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical challenges. One example is, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you must get notified right away. This aids you resolve problems fast, often right before people even detect.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it brings about actual damage.
As your application grows, site visitors and knowledge improve. Without the need of checking, you’ll miss indications of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In brief, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it really works properly, even stressed.
Ultimate Views
Scalability isn’t only for big firms. Even small applications need a powerful Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you can Create applications that develop efficiently without the need of breaking under pressure. Start off small, Feel major, and Develop sensible.